Showing posts with label ES6. Show all posts
Monday 10 June 2019
How to Build a Paint App!
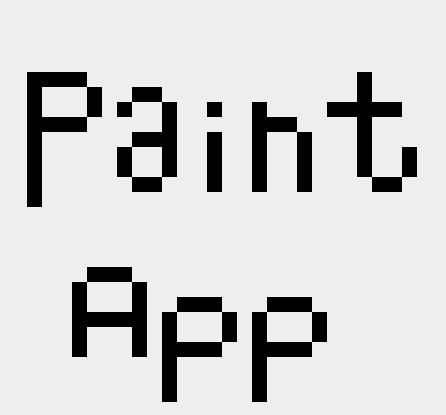
In this tutorial you will learn how to create a simple paint app coded in ES6. All you need to understand this tutorial in some basic knowledge of ES6 and a few months of coding experience in Javascript/ES6 and you are good to go!
Now this is just a simple “Paint App” but upon this same logic a lot of complex software can be developed.
Go through the following exercise, keep it simple, and take it as it comes:
1) Use javaScript/ES6 to generate HTML & CSS.
2) Build a “Paint App” with the ability to alter resolution by mutating the app.resW & app.resH variables.
3) The display should represent a 2d array named matrix.
4) Compound loops should be used to iterate through the matrix/multidimensional-array.
Here is the code we have:
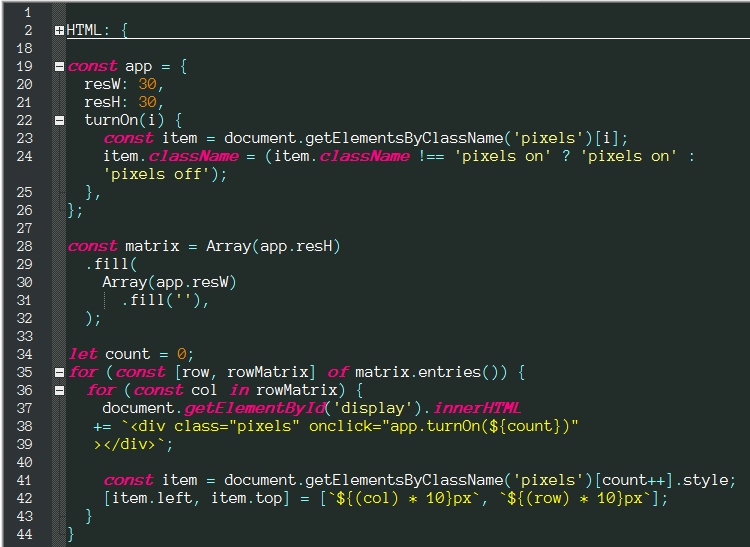
The HTML part is:
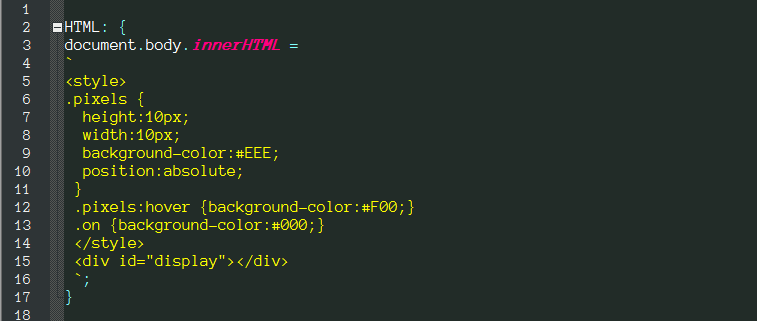
To view it in the browser:
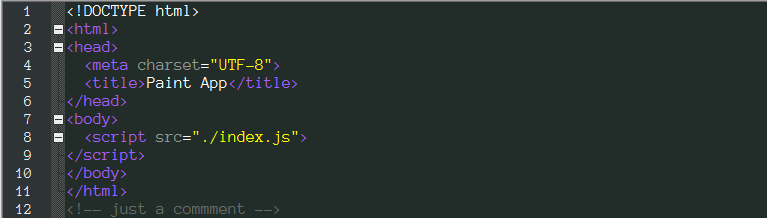
Now let me narrate each line of code so we can learn it better:
First of all there is the HTML label, it covers most of the HTML & CSS generation process of our code.
There is a class named pixels which belongs to each individual pixel on our canvas, it makes our pixels square shaped. Now in modern day an actual pixel is one of those really small squares on the screen, however back in the day those squares used to be gigantic like the ones in our app, our browser may crash if we try to generate a 1px², 600px*800px canvas, so instead we are generating a 10px²,30px*30px canvas.
There is a hover pseudo-class on pixels, this highlights (in red) the pixel to show the user which pixel their identifier/cursor is targeting.
There is a class named on which changes the color of a white pixel to a black pixel giving the user the ability to draw and write on the canvas with black color.
There is a divider with id “display” this will clench all our pixels within itself. This concludes the HTML label portion of our code.
Then on line #19 we have an object named “app”, in this object we are plotting some vital policies of our app, we have the width and the amount of pixels assembled in each row of pixels in our app’s canvas established in the resW property, and we have the height and the amount of pixels assembled in each column of pixels in our app’s canvas established in the resH property.
Finally we have the method that toggles a pixel between black and white colors so we can draw upon our canvas. To this point we are just storing data that is a prerequisite to spreading out a mutable canvas.
Finally we have the method that toggles a pixel between black and white colors so we can draw upon our canvas. To this point we are just storing data that is a prerequisite to spreading out a mutable canvas.
Then on lines #29 to #33 we have a variable named “matrix”, this is a record of each pixel in our canvas, if we ever need to export our drawings into a separate file to save them we will actually be saving a 2-dimentional matrix of pixel data. This code narrates like this “Declare a variable named matrix, in this variable we have an array with (resH) amount of members, within this array all members are arrays, each of the latter array has (resW) amount of members, each of these members are an empty-string”.
Now from line #35 to #47 we have a two-way loop that extracts each pixel data from our matrix and directs it towards display on lines #39 to #41; Now on lines #43 to #46 we are placing/spreading each pixel to its proper place. The row & col variables on lines #36 & #37 are index numbers and are used as co-ordinates for pixels. The code narrates like this “Extract the 1st pixel from the matrix and spread it on (#display) canvas, give this pixel the class `pixels` and the ability to toggle between black and white colors when clicked; place this pixel on co-ordinates (x, y) where x equals 10 times its vertical placement in the matrix and y equals 10 times its horizontal placement in the matrix”.
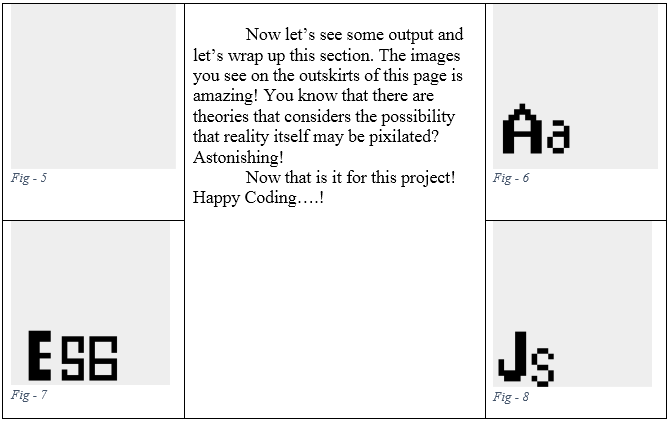
This code is taken from the #1 best-seller book Simply ES6 By Ray Voice.
Simply ES6 is a great book for you whether you are a few months or few years into your web-development training. This book will save you years and years of frustrated lessons.
The lessons are well structured, each section begins with a brief introduction, followed by tons of examples to follow and play with; and each chapter ends with a practice project explained in extensive detail, followed by a summary of that chapter to really condense the skills learned.
If you love JavaScript then this book is for you! If you want to master JavaScript then this book is for you!
Simply ES6 is a great book for you whether you are a few months or few years into your web-development training. This book will save you years and years of frustrated lessons.
The lessons are well structured, each section begins with a brief introduction, followed by tons of examples to follow and play with; and each chapter ends with a practice project explained in extensive detail, followed by a summary of that chapter to really condense the skills learned.
If you love JavaScript then this book is for you! If you want to master JavaScript then this book is for you!
Get yourself a copy of the #1 new release & #1 best-seller from Amazon Now!
Subscribe to:
Posts
(
Atom
)